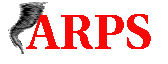
|
ARPS Local Developers' Corner
ARPS Official Code Update, Version Control and Release Policy
ARPS Code Update, Version Control and Release Policy
24 Sep 1998
DRAFT
1. Official releases shall consist of either
(a) a complete (3-digit, tertiary) version, or
(b) an update to a complete version, identified by a fourth digit.
2. Official releases shall never be modified. The date of the release shall be noted in the HISTORY file. Official releases shall be announced by email and by a web posting.
3. Officially releases shall be maintained in the following
locations:
(a) the ftp server (tarred/gzipped; ftp.caps.ou.edu), and
(b) a disk accessible from both the Origin and the RS/6000 cluster (untarred;
last 6 months only; origin:/official).
Between complete official releases of ARPS, official updates
will be released as needed. They are intended primarily to address bug fixes
or other simple changes. The updates will not necessarily be tested. They will
contain all files modified since the previous complete official release, and
only those files.
As an example, 4.9.1.update.2 will contain all files modified since 4.9.1 was released. (Thus there is no need to refer to 4.9.1.update.1.) A script will be provided to move the updated files to their proper location while preserving any existing files. The updated version may be referred to as 4.9.1.2.
4. A suite of basic tests will be performed before a complete version is released. These tests currently consist of several idealized tests (e.g., May 20, symmetry, density current, Beltrami flow) and a real data case [TBD]. Any variance in test results (due to changes in model algorithms, etc.) must be noted in the HISTORY file and be approved by the code's primary author.
It is the developer's responsibility, however, to perform tests that demonstrate that the effectiveness of the proposed changes. These tests should be run before the proposed changes are submitted. Once a "work" version is created, but before any official release, the maintainer may request that the developer re-run such tests.
Preliminary, untested ("beta") versions may sometimes be made available for testing by the Development Group. They shall be clearly identified as such (e.g., ".wrk"), and will be intended for testing only, not operational use. (Users are advised not to access files in the maintainer's work directory except by special arrangement.)
5. Developers shall request changes by sending email to the ARPS Development Group (arpsdev@listserv.ou.edu). (Mail sent to this address will automatically be sent to all members of the group and will be archived on a web page [TBD]. Thus, members may simply reply to the email without referring to the web page.) The developer shall describe
- the specific code change(s) that are to be made;
- tests that have been performed that demonstrate the accuracy of the proposed changes.
- whether the change should be made immediately as an update or whether it can wait till the next complete release;
- any other relevant information.
At the time the request is made, the maintainer will post an estimated time for making this change. He or she will also notify the group when the change is implemented in an officially released version. The purpose for this procedure is to avoid miscommunication and to involve more people in the development process.
ARPS Coding Standard
ARPS Version 5.0 Coding Standard in Fortran 90
7/30/1998
Updated 8/7/1998
General rules and conventions
- Use Fortran 90 free format;
- Use IMPLICIT NONE in all program units;
- Use MODULES to pass global variables (not grid size-dependent data arrays)
among program units, avoid using COMMON;
- Pass all arrays as subprogram arguments, except for predefined fixed-sized
data arrays. No array whose size depends on the grid size should be passed
via common blocks. In fact, the use of common blocks should be entirely avoided;
- All global parameters should be set at the initialization stage;
- Do not use EQUIVALENCE;
- Start comment lines with exclamation marks !. When the comment line is a
stand along line, it should start from the first column. Comments can also
follow executable statements;
- Use upper case for ALL Fortran key word, e.g., SUBROUTINE, DO, IF, END,
.AND., .OR., IMPLICIT NONE, CALL, WRITE, READ, OPEN, MODULE, PROGRAM, RETORN,
STOP, END;
- All variable names and program unit names should be in lower case;
- Use new logical operators (e.g., >, <, ==, /=) instead of old ones
(e.g., .gt., .lt., .eq., .ne.) in new code;
- Use !------------------------------------------------------------------------
to separate comments from executable source. The length of the separate is
72 characters;
- Use blank lines to separate statement blocks to improve code readability;
- Limit the executable statements to within 72 columns. This is to optimize
the use of screen and printing space. Comment lines can go beyond 72 columns
but preferably within 100 columns;
- Place the continuation marker '&' in column 73;
- Try to place input, output and work arrarys are separate lines in the actual
and dummy argument lists;
- Define all input and output variables found in the argument list of all
program units. Declare the INTENT of all arguments;
- Start and end a program unit in the first column and indent the program
body by two spaces;
- Indent successive nests (e.g., DO loop, IF nest) by two spaces;
- Do not use TAB in source code because editors and printers may have different
definitions of TAB length;
- Use descriptive and unique names for variables and subprograms, so as to
improve the code readability and facilitate global string search (e.g., using
grep under UNIX);
- Loops should be ordered k (for z), j (for y), i (for x), where i is in the
innermost and k the outermost;
- Use the same name for the same variable across all program units;
- Enforce SI unit in all new code;
- Array dimensions should be in the following order: x (east-west), y (north-south),
z (vertical), t (time level). Additional dimensions can be used for .e.g,
the number of scalar variables, and/or the grid/domain.
- Try to limit variable and subprogram name lengths to and under 12 characters;
- Boundary condition codes, especially those for the lateral boundaries, should
be isolated and placed in separate subroutines, which should be grouped together.
This is to ease the parallelization on distributed-memory processors via domain
decomposition;
- I/O should also be isolated into separate I/O subroutines;
- All loops should be vectorizable in the x and y direction whenever possible.
I.e., the operations for grid points in the horizontal directions should be
independent. This is required by horizontal domain decomposition, and also
ensures code efficiency on vector processors;
- Use Fortran 90 TYPE to define data stuctures for multiple nested grids;
- Avoid using Fortran 90 POINTER whenever possible;
- All arrays whose size depends on the grid size should be dynamically allocatable,
i.e., they should not be declared as fix-sized arrays. This is so so that
the entire model solver can be called for domains/grids of varying sizes;
- Use automatic arrays only when essential, which usually means at the upper-levels
of programs;
- Temporary (work) arrays should be shared across subprogram levels as much
as possible to minimize the total memory usage. For example, suppose a program
has ten levels of subroutine calls, and at each level 10 temporary arrays
are declared as automatic arrays. Unless arrays whose contents are not needed
after the subroutine calls are explicitly deallocated before the calls (often
reallocated again after the calls), the total temporary storage required by
this program is the sum of all 100 arrays. Significant saving can be gained
if these arrays are shared across the program levels, which means that they
are passed down to the lower levels through the argument list;
- The ranks and/or dimension sizes of actual and dummy arguments can differ
only when it is necessary to pass a subset of the actual array to the dummy
array. E.g., passing the horizontal slice of 3D array Ua(nx,ny,nz) at the
2nd grid level to a 2D dummy array is allowed in
CALL sub(nx,ny, ua(:,:,2)). Inside subroutine sub, dummy array Ud should
be defined as REAL :: ud(nx,ny), whose shape should match the subset of array
Ua passed in. Passing array of different ranks and/or dimension sizes to the
dummy array should avoided if at all possible.
- The code should be moderately modular. Repeated tasked should be done inside
subroutines, function or modules;
- Extremely short subroutine (e.g. those that do only one loop operation)
should be avoided because they are often inefficient. Remember that extra
memory access (e.g., assignment of values to intermediate arrays) can be expensive,
on both conventional memory architecture platforms (e.g., Cray J90) and cache-based
multi-level memory architectures (e.g., Origin 2000);
- Avoid very long program units. Limit the length to under 500 lines;
- Use intrinsic functions (e.g., MAXVAL, MAXLOC) whenever possible;
- Use array syntax operation only when the operation is on the entire array
or when the statement is very short. An example for the former case is a(:)=b(:)*c(:)
where a, b and c are 1-D arrays. For the latter, a(1:nx-1)=b(1:nx-1) is okey,
but statement like a(1:nx-1)=b(1:nx-1)*(c(2:nx)+d(1:nx-1)) should be avoided
becasue it is more prone to coding errors, and is not friendly to MPP pre-processors/translators.
Use names for the DO loops when necessary.
- Write no more than one statement on each line;
- Be Y2K compliant;
Rules and conventions used in ARPS subroutines
Subroutine layout:
Header declaration
SUBROUTINE subname(input_argument_list, output_argument_list, work_arrays)
Purpose
Author, date, and modification history
Use module statement
IMPLICIT NONE statement
Declaration of variables in argument list with INTENT together with variable
definitions including units
Local variable declaration
PARAMETER statements
Executable code
CONTAINS statement
Internal subprograms or module subprograms
END SUBROUTINE subname
Obsolescent Fortran features to avoid:
- Fixed form;
- Data type DOUBLE PRECISION;
- Separate DIMENSION statement;
- Old type specification without ::;
- Old PARAMETER statement (e.g., PARAMETER(nx=10));
- Old DATA statement, in fact DATA statement can be avoided all together;
- Labeled (using numbers) DO loop;
- CONTINUE statement;
- DO WHILE statement;
- Real value as DO loop variable;
- Arithmetic IF;
- ASSIGN and PAUSE statement;
- ERR and END in I/O statement, use IOSTAT instead (debatable);
- String format with H;
- Statement function, use internal procedure instead;
- Use of type specific intrinsic functions (e.g., amax, dmax, use max in all
cases);
- ENTRY statement;
- Alternate RETURN
Example code
Subroutine solvq.f90 is an example written according
the above standard.
About ARPS CVS
ARPSDEV Mail List
arpsdev@listserv.ou.edu, an autosubscribing mailing list, is set up
for local developers and testers of ARPS to post code integration requsts,
and report the status of the merged code. All mail postings are archived at
http://lists.ou.edu/archives/arpsdev.html
Only request send to arpsdev@listserv.ou.edu
will be accepted. Subscription to the mail listing
is not open to all. Send request for adding yourself to mxue [at] ou . edu.
ARPSTESTING Mail List
Where messages sent to arpstesting@lists.ou.edu
are achived. This archive is intended for internal use only. Send request
to mxue [at] ou . edu if you think you should
be included.
|
|